What is the best way to learn React JS?
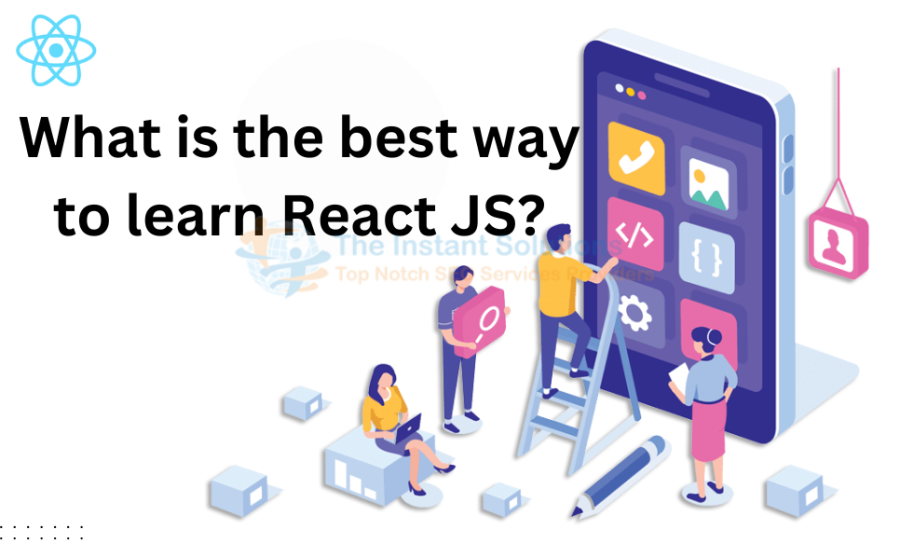
What is React JS?
React JS is a widely used open-source JavaScript library primarily used for the construction of UIs, such as SPAs that update information on the screen without reloading the entire page. Originally developed by Facebook and is now maintained by Facebook and an extensive community of developers. Let's discuss what is the best way to learn React.js.
What is the best way to learn React.js?
Step 1: Master the Basics of Web Development
Before diving into learn React, it’s essential to have a solid understanding of web development fundamentals. This includes HTML, CSS, and JavaScript, which form the backbone of any React application.
- HTML & CSS: Understand the structure and styling of web pages. Learn about semantic HTML and responsive design.
- JavaScript (ES6+): Become familiar with modern JavaScript features like arrow functions, destructuring, template literals, and modules.
- Array Methods: Map, filter, and reduce.
- Asynchronous Operations: Learn how to handle asynchronous operations using async/await.
Related topics you may enjoy: What is Vue.JS? An Introduction to Vue Framework
Tools:
- Use a code editor like Visual Studio Code for a better development experience.
- Practice building simple web pages with dynamic components, such as a form or a gallery.
Step 2: Set Up Your Development Environment
To start with React, you must set up your development environment.
Install Node.js and npm:
- Visit the Node.js website and install the latest version.
- npm (Node Package Manager) is bundled with Node.js and will help you install React and other dependencies.
Create a React Project:
Using Create React App:
bash
npx create-react-app my-app cd my-app npm start
- Using Vite (a faster alternative):
bash
npm create vite@latest my-app --template react cd my-app npm install npm run dev
Practice:
- Open the App.js file and modify it to display "Hello, React!" to verify that everything is working.
Step 3: Learn React Core Concepts
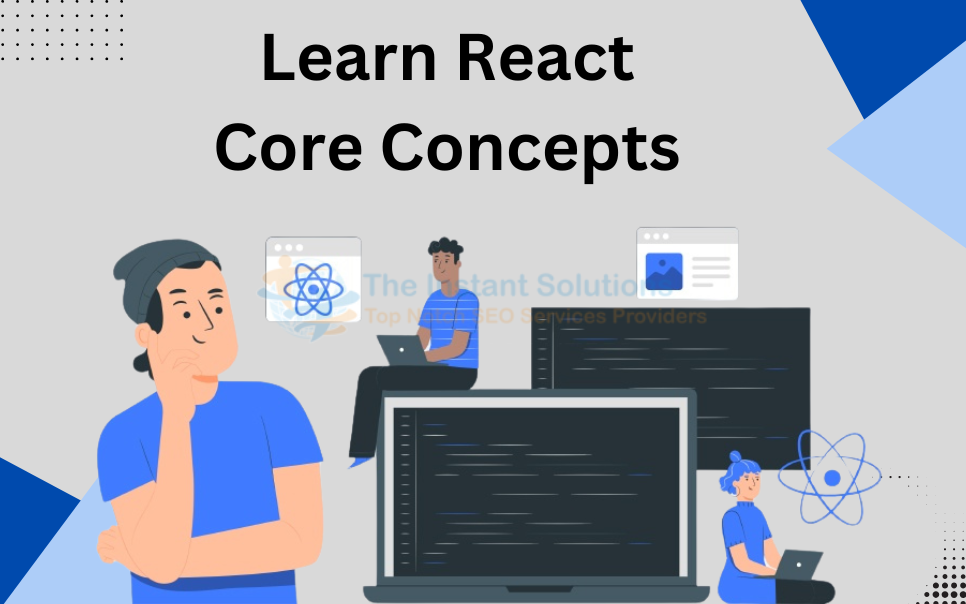
React is built around a few key concepts. Understanding these is crucial to mastering React.
Core Concepts:
- Components: Learn how to create and use reusable UI components.
- JSX (JavaScript XML): JSX allows you to write HTML-like code within JavaScript.
- Props: Props are used to pass data between components.
- State: State allows you to manage dynamic data that changes over time.
Practice:
- Build a To-Do List App where you can add, remove, and mark tasks as complete. This project will help you understand components, props, and state.
Step 4: Exploring React Hooks
React introduced Hooks to make functional components more powerful and reduce the need for class components.it encourages the reader to learn React JS. The most common hooks are useState, useEffect, and useContext.
Key Hooks to Learn:
- Use State: Manage state in functional components.
- Use Effect: Handle side effects like fetching data or updating the DOM.
- Use Context: Share data across components without prop drilling.
Practice:
- Build a Weather App that fetches weather data from an API using the use effect to update the UI dynamically based on the fetched data.
Step 5: Understand React Router
React Router is a library that allows you to handle navigation in your application. It enables you to build multi-page apps with React.
Steps to Use React Router:
Install react-router-dom:
bash
npm install react-router-dom
- Define routes for different pages like Home, About, and Contact.
Practice:
- Build a Portfolio Website with different pages (e.g., home, projects, about, contact) using React Router to manage navigation.
Step 6: Learn React.js Advanced State Management
For larger applications, managing the state becomes more complex. React provides several ways to handle state efficiently.
Options for State Management:
- Context API: Good for sharing state globally within the app, especially in smaller apps.
- Redux: A powerful state management tool for larger, more complex apps.
- Zustand: A lightweight alternative to Redux for state management.
Practice:
- Build an E-commerce App where you manage a shopping cart across multiple pages using either Context API or Redux.
Step 7: Styling Techniques in React
Styling in React can be done in several ways, depending on your project needs.
Styling Approaches:
- CSS-in-JS: Libraries like Styled Components or Emotion allow you to write CSS directly inside JavaScript.
- CSS Frameworks: Use frameworks like Tailwind CSS or Bootstrap for quick, responsive designs.
- Component Libraries: Libraries like Material-UI offer pre-built UI components.
Practice:
- Style your To-Do List App or Portfolio Website using your preferred styling method.
Step 8: Optimize and Deploy Your React App
Once your React app is complete, it’s time to optimize and deploy it.
Performance Optimization:
- Memoization: Use React. memo, useMemo, and use callback to prevent unnecessary re-renders and improve performance.
- Code Splitting: Implement lazy loading using React.lazy and Suspense to split large components into smaller ones that load on demand.
Deploying Your React App:
- Vercel: Simple deployment for React apps with automatic GitHub integration.
- Netlify: Another popular choice for deploying React apps.
- GitHub Pages: For static React apps.
Practice:
- Deploy your To-Do List App or Portfolio Website to platforms like Vercel or Netlify.
Step 9: Build Real-World Projects
The best way to reinforce your React skills is by building real-world applications. This will challenge you to use multiple React concepts and techniques in a single project.
Project Ideas:
- Chat App: Use Firebase or Socket.io for real-time communication.
- Task Tracker: Add CRUD operations and authentication using Firebase or Auth0.
- Movie App: Fetch and display movie data from a public API like TMDB.
Conclusion
Mastering React JS takes time and practice. Start small with simple projects and gradually increase the complexity as you learn React.js. Keep building, experimenting, and seeking out resources to continue improving your skills. React’s rich ecosystem and vibrant community provide endless growth opportunities.
As you grow more comfortable with React, you can dive into more advanced topics like Server-Side Rendering (SSR) with Next.js, Static Site Generation (SSG), or explore TypeScript with React for type safety.