What is next.js? A Complete Beginners Guide 2025
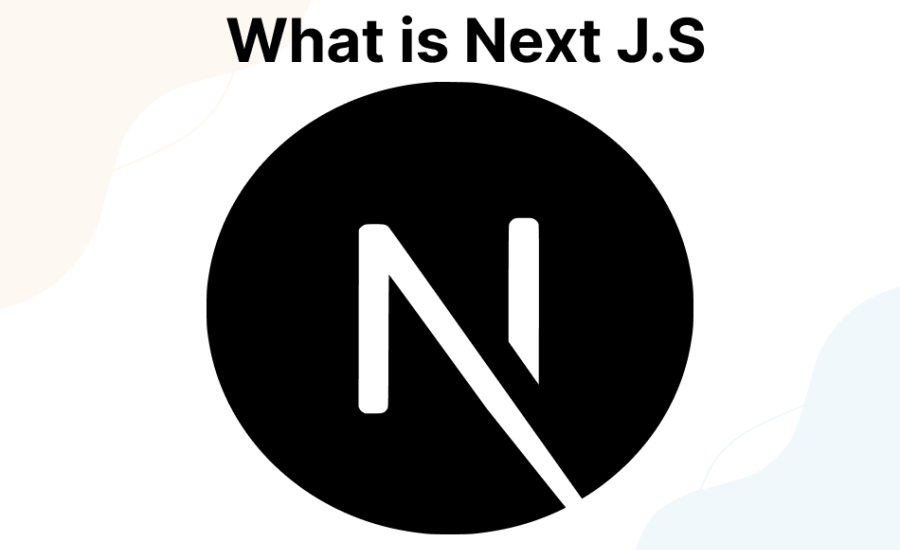
What is Next JS? A Complete Guide
Next.js is a React-based meta-framework, that is built on top of React to provide more structure and additional features such as server-side rendering, routing, bundling, and more. It is commonly used to build SEO-friendly, high-performance web applications with ease. Built on React, Next.js makes front-end development easier by offering features like server-side rendering (SSR) and static site generation (SSG). It also includes built-in CSS and JavaScript bundling to optimize performance Next.js is highly scalable and SEO-friendly, which makes it an excellent choice for modern web applications.
To get started with Next.js, you’ll need to install and set it up on your system.
Relate topics you may enjoy: What is React.js?Top examples
Create a next.js app
To begin with Next.js, let's create a new app using the CLI. This will generate a set of starter files for you.
1. Create the app
Run the following command to initialize your app:
$ npx create-next-app color-pages
$ cd color-pages
2. Start the development server
To launch the development server with hot reloading, use:
$ npm run dev
3. Set up the color data
Run the following commands:
mkdir data
touch data/colors.json
Here's the structure for the colors.json file:
// data/colors.json
[
{ "name": "Illuminating", "hex": "#F5DF4D" },
{ "name": "Classic Blue", "hex": "#0f4c81" },
{ "name": "Living Coral", "hex": "#FA7268" },
{ "name": "Ultra Violet", "hex": "#5f4b8b" },
{ "name": "Greenery", "hex": "#88b04b" },
{ "name": "Rose Quartz", "hex": "#F7CAC9" },
{ "name": "Marsala", "hex": "#B57170" },
{ "name": "Radiant Orchid", "hex": "#b067a1" }
Routing
Next.js handles routing automatically: files in the pages folder become routes. For example, about.js will correspond to the /about route. The special case is index.js, which maps to /.
To dynamically create pages for each color, we'll use dynamic routes. We'll create a file called [color].js inside the pages folder, which will allow us to render a unique page for each color (like /Classic Blue, /Ultra Violet, etc.).
4. Create a dynamic route for color pages
Run:
$ touch pages/[color].js
getStaticPaths
We need to create the getStaticPaths() function to generate static pages for each color. This function will loop through the colors and dynamically generate paths. For example, /Marsala will render the page showing details about the "Marsala" color.
Here’s how the getStaticPaths() function looks:
// [color].js
// import the colors array
import colors from '../data/colors.json'
export async function getStaticPaths() {
// loop through the colors array
const paths = colors.map(color => ({
// return an object with params.color set to the color's name
params: { color: color.name }
}))
return { paths, fallback: false } }
the paths generated will look something like
// [
// { params: { color: 'Marsala' } },
// { params: { color: 'Illuminating'} }
// ...
// ]
getStaticProps
Now, let's define getStaticProps(). This function will fetch the data for the specific color based on the route parameter (params.color). For example, for /Marsala, it will return the data for the "Marsala" color.
// [color].js
export async function getStaticProps({ params })
{
// find the info for just one color
const color = colors.find(color => color.name === params.color)
// return it in the necessary format.
return { props: { color } }
}
Page Template
Now, let's create the React component to display the color page. The color object from getStaticProps will be passed as props to the component. We can use the hex value to set the background color of the page and render the color name.
// [color.js]
export default function Color({ color }) {
return <div className='color-page' style={{ backgroundColor: color.hex }}>
<h1>{color.name}</h1>
</div>
}
Styles
Here's an updated global.css for better styling:
/* global.css */
html,
body, #__next, .color-page {
padding: 0;
margin: 0;
height: 100%;
width: 100%;
top: 0px;
position: absolute;
display: block;
font-family: -apple-system, BlinkMacSystemFont, Segoe UI, Roboto, Oxygen,
Ubuntu, Cantarell, Fira Sans, Droid Sans, Helvetica Neue, sans-serif;
}
.color-page {
display: flex;
justify-content: center;
align-items: center;
}
Link Component
Next, we'll update index.js to display the list of colors on the homepage. We’ll use Next.js's link component for client-side navigation between pages, and the Head component to manage meta tags.
// index.js
import Head from 'next/head'
import Link from 'next/link'
import colors from '../data/colors.json'
export default function Home() {
return (
<div>
<Head>
<title>Colors!</title>
<meta name="description" content=" App that displays pretty colors to learn Next!" />
</Head>
{colors.map(color => (
<Link href={`/${color.name}`}>
<h2>{color.name}</h2>
</Link>
))}
</div>
)
}
SSR vs. SSG
What we've built so far is a statically generated Next.js app (SSG). This means the HTML for each page is generated during the build process, so it's fast and cacheable by a CDN. However, this method doesn't automatically update content unless you rebuild the app.
If you need content to update dynamically based on user requests (e.g., fetching data from an API), you can use server-side rendering (SSR). With SSR, Next.js renders the page on the server for each request.
To switch to SSR, you can replace getStaticProps() and getStaticPaths() with getServerSideProps():
export async function getServerSideProps({ params }) {
//Request to get data about the color via our API
const res = await fetch(`http://www.color-api.com/${params.color}`)
const color = await fetch.json()
// return the data as props that will be passed to the Color component
return { props: { color } }
}
Deployment
Once you're done, you can deploy your app. AWS Amplify supports both SSR and SSG without extra configuration.
For a statically generated app, modify your package.json to include the next export command:
"scripts": {
"dev": "next dev",
+ "build": "next build && next export",
"start": "next start"
},
Push your code to your Git repository, create an AWS account (if you don’t have one), and navigate to the Amplify Console. Follow the steps to link your GitHub repo, configure build settings, and deploy.
Conclusion
Next.js offers an amazing developer experience which makes it easy to build fast, performant websites with minimal effort. With excellent documentation and useful built-in features like static site generation, server-side rendering, and routing, it's a great choice for building modern React apps.